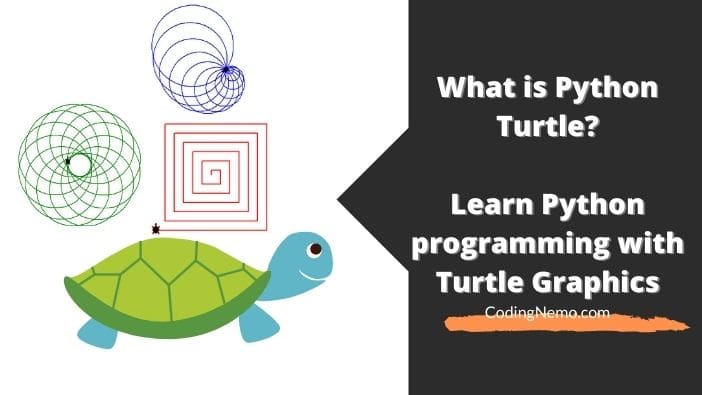
This post is part of the upcoming Python for kids series. The series will provide kids or beginners the easiest and fun way to learn Python programming.
Looking to download the FREE Python Turtle worksheet? Go HERE
What is Python Turtle?
Python Turtle refers to the capability of the Python programming language to create Turtle graphics. It is a pre-installed Python library that provides programmers the ability to draw different types of shapes, colors, and images.
Drawing Python Turtle graphics offers new coders an excellent starting point to get involved in python programming.
In this blog post, you will learn about how to start drawing turtle graphics with python programming.
So, the next question, what is turtle graphics?
What is Turtle Graphics?
Turtle graphics was derived from a robot of the same name. It was introduced as part of the classical LOGO programming language back in 1967. LOGO is an educational programming language that was specifically designed for children.
LOGO programming features an on-screen cursor or “turtle” that draws lines and shapes as it moves around the computer screen. A programmer controls the cursor by writing LOGO codes.
What are the benefits of learning turtle graphics?
Why do I recommend kids or new coders to learn turtle graphics? I was introduced to turtle graphics when I first started learning LOGO programming. Even after years, I still appreciate the values and fun the turtle graphics offered.
There are many benefits of learning turtle graphics, let’s look at a few of them here:
Turtle graphics programming is engaging.
Kids control the cursor (or the Turtle) by writing codes. It is interactive, kids receive feedback on their codes instantly. This is very similar to controlling a robotic toy, but this time is on the screen. Drawing Turtle graphics makes learning to code fun.
Turtle graphics programming is easy to learn
Whether it is with LOGO or Python, it is very easy to pick up turtle graphic programming. Most middle school kids can understand the fundamentals of the language and start playing with it within a day.
Turtle graphics are beautiful, it is a great way to introduce coding to kids
Turtle graphics programming is an excellent channel to introduce programming to kids and at the same time motivate them to write code. The beautiful graphics attract them and show kids what they can do with writing computer codes.
Quick Python Development Environment setup
Python is free to use and develop. In this section, I will give you a quick guide to install Python and how to use the development environment IDLE to write code.
I will use windows installations as an example here.
There are two methods to install Python on the Windows system.
- Install Python App package from Microsoft Store, OR
- Install Full Python package downloaded from Python.org
If you are new to Python programming and you aim to focus on learning the language rather than building a complex python project, I would suggest installing Python with the Microsoft Store package.
Microsoft Store package is an easily installable Python interpreter that is intended for students. (Source from Python.org)
There are some limitations with the Microsoft Store Python package, but those will not affect our learning experience, and the installation process is much simpler for beginners.
For now, we are focusing on learning.
Install Python from Microsoft Store
#1 Click on the Windows Start button and type in “Microsoft Store”. This will bring you the Microsoft Store app. Open the app.
#2 In the Microsoft Store, type “Python” in the search box
Alternatively, you can click on this direct link to Microsoft Store.
#3 Select the Python version you would like to install. For learning, I choose the latest version.
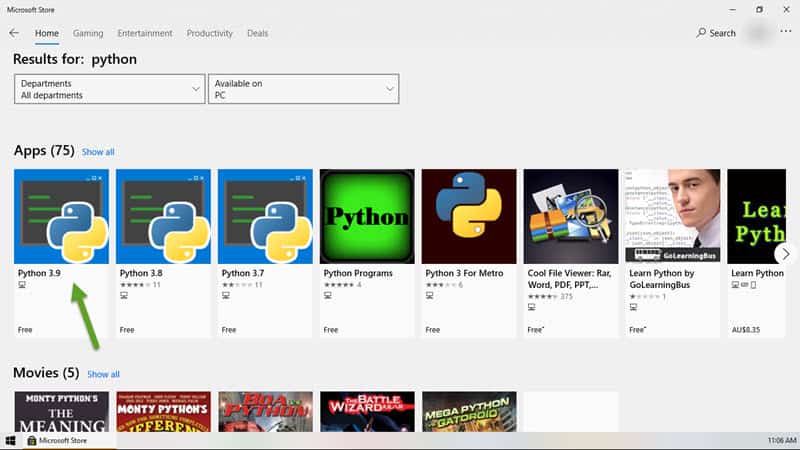
#4 It is important to make sure the Python selected is by “Python Software Foundation”. And it is always FREE to download. (If you are asked to pay for it, you are looking at the wrong package).
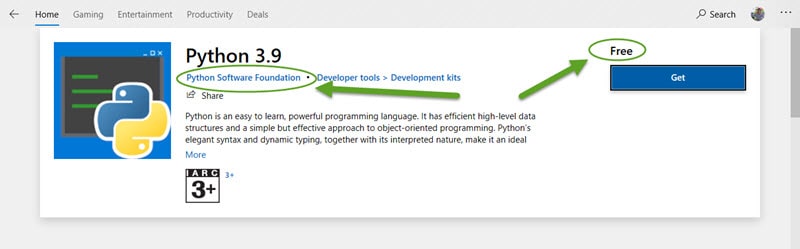
#5 Click on Get and then Install button to start the installation.
#6 That’s it. You have successfully installed Python on your Windows PC. Now, you have access to Python pip, and IDLE.
Learning to code with Python IDLE
Python IDLE stands for Integrated Development and Learning Environment. It is a tool for programmers to write and execute Python code.
To start IDLE in Windows, click on Windows Start Button and type “IDLE”.
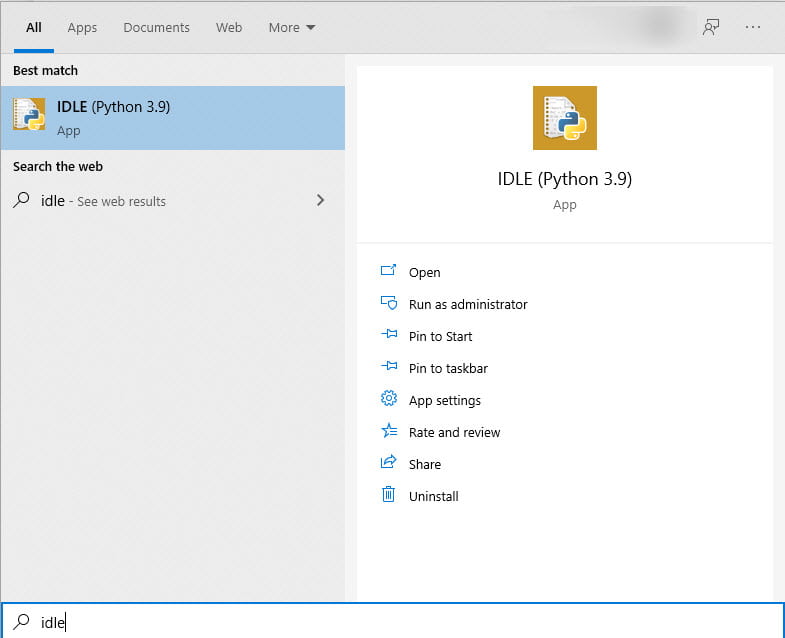
With Python IDLE, you can create a new python file, open an existing Python program, or execute a python program.
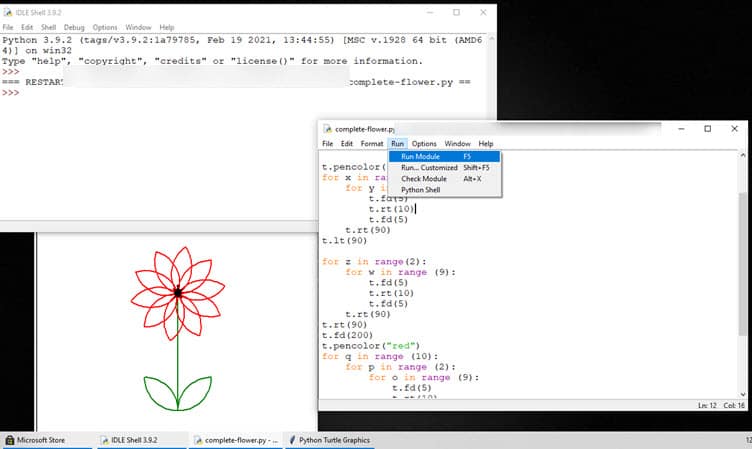
Run your first Python Turtle commands
Let’s start playing around with the basic Python Turtle commands in the interactive IDLE window.
To get started with Python Turtle, we need to import the Python Turtle library into our development environment.
A library is a set of functions and methods that you can reuse to create python programs.
In the IDLE, type “import turtle” in the command line.
>>> import turtle
Next, we want to see the turtle graphic in real-time while we code. To achieve this, we need to enable turtle screen:
>>> t_screen=turtle.getscreen()
“t_screen” is a variable that represents your turtle screen. It could be any name you like. Once you have executed the getscreen() commands, a new window would appear with a small, black triangular shape in the middle. That’s your “turtle.
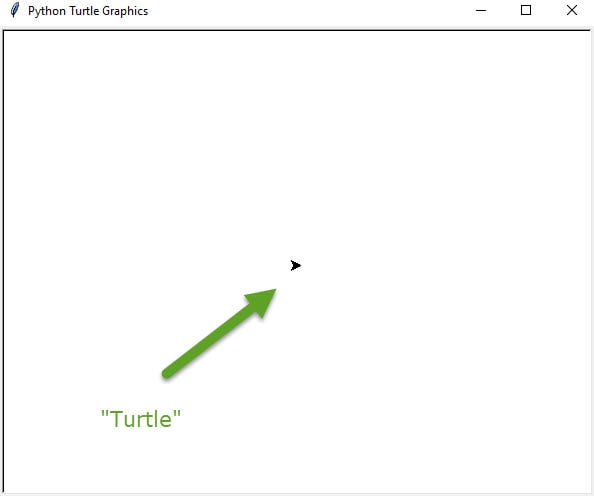
Next, we need to initialize a variable that represents the turtle.
>>> MyT = turtle.Turtle()
“MyT” is a variable that represents Turtle. We will assign actions or attributes like color and size to it throughout our program.
That’s it. We now have the turtle screen and turtle ready. In the next section, we will look at some basic python turtle commands.
Basic Python Turtle Command
Python offers a module that reimplements the classical Turtle Graphics module with Python language.
It keeps the merits of the classical turtle graphic module and tries to be nearly 100% compatible with it.
Let’s look at the list of basic Python Turtle commands that kids or new coders can start playing with it.
The Direction of Turtle
We can move or rotate the Turtle to specific angles or direction by using the commands below:
- left() or shortened command lt()
- right() or shortened command rt()
- forward() or shortened command fd()
- backward() or shortened command bk()
Python Commands | Explanations | Example |
---|---|---|
left() | Rotate the Turtle to the left with a specific angle. | left(90) – rotate 90 degrees to the left from its current position |
right() | Rotate the Turtle to the right with a specific angle. | right(90)- rotate 90 degrees to the right from its current position |
forward() | Move the turtle forward with a specific number of pixels | forward(100) – move the turtle forward 100 dots/pixels. |
backward() | move the turtle backward with a specific number of pixels | backward(100) – move the turtle backward 100 dots/pixels. |
As the turtle moves, it will draw a line on the computer screen. Remember, a dot or pixel on the computer screen is really small, so it might be hard to see the line if the turtle only moves 1 or 2 pixels. But you will see the line when it moves about 100 pixels.
Let’s use the commands to draw a square on our turtle screen, shall we?
>>>MyT.forward(100)
>>>MyT.left(90)
>>>MyT.forward(100)
>>>MyT.left(90)
>>>MyT.forward(100)
>>>MyT.left(90)
>>>MyT.forward(100)
“MyT” is the variable that represents the turtle. We define the variable in the earlier part of the article.
The commands above are telling the Turtle to move forward 100 pixels, then turn 90 degrees to the left from its current direction. By Repeating the same action 4 times, we have a perfect square drawn on the computer screen.
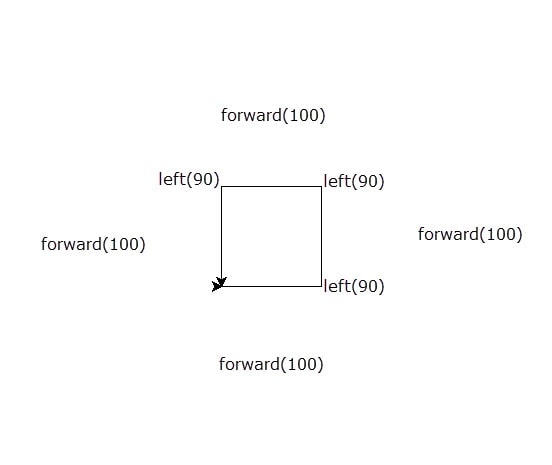
We can draw the square in a faster way by using the Looping concept.
Looping is the programming concept that repeating a set of instructions until certain conditions are met.
Let’s use the command below:
>>> for x in range (4):
MyT.forward(100)
MyT.left(90)
We just did the same job with only 3 lines of codes!
Here is how it works:
x is the variable that represents how many times we want the actions to be repeated. In our example, we want the same actions to be repeated 4 times.
x started from 0, and it is increased by 1 after each iteration. So it goes like this:
- x=0, perform action, x +1
- x=1, perform action, x +1
- x=2, perform action, x +1
- x=3, perform action, x +1
- x=4, stop
So now we have the basic concepts of Python turtle, here is a list of commands that you can explore further:
You can run these commands in by entering them in the similar way as
>>>MyT.forward(100)
or, if you did not declare any variable, you can run the command like this:
>>>turtle.forward(100)
Python Turtle Commands | Explainations |
---|---|
turtle.circle(50) | Draw a circle with radius=50 pixels |
turtle.dot(50) | draw a dot with diameter = 50 pixels |
turtle.bgcolor(“blue”) | set the background color of the turtle screen to “blue”. |
turtle.pencolor(“red”) | set the pen color (line color) of the turtle to “red” |
turtle.width(50) | set the width of the turtle’s pen (line width) to 50 |
turtle.shape (“turtle”) | set the shape of the cursor to the turtle. There are 6 choices of Turtle shape: Turtle, Arrow, Triangle, Circle, Square, Classic. |
turtle.color(“red”) | set the color of the turtle shape to “red” |
turtle.speed(1) | set the speed of the turtle (drawing speed) to 1 |
turtle.penup() | lift the turtle pen up, so when it moves it does not draw on the screen. |
turtle.pendown() | this command needs to be used after penup(). It put the turtle pen back. The turtle will start drawing lines as it moves. |
turtle.clear() | Clear the screen. All drawings on the screen will be removed. |
For more Python commands, you can check out Python Turtle and Screen Methods.
3 examples of turtle graphics for kids
Let’s look at 3 examples of Python Turtle graphics. These are simple graphics meant to show you the basics of Python Turtle programming.
Feel free to change and explore the functionalities of the Python turtle library.
Example 1, Drawing 3 Stars and fill with colors
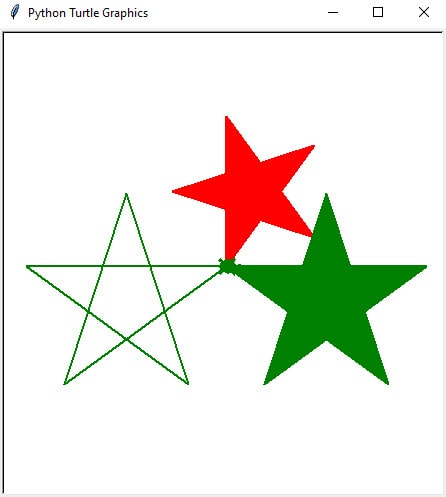
Python Turtle Code:
import turtle
t = turtle.Pen()
t.speed(1) #drawing speed
t.shape("turtle")
t.pensize(2)
t.lt(90) #to make sure turtle is facing north at the start
size=150 #star size
t.color("red") #set the cursor/turtle color to "red"
t.begin_fill() #fill the 1st star red
for x in range (5):
t.fd(150)
t.rt(144)
t.end_fill()
t.lt(90)
t.color("green") #set the turtle color to "green"
for x in range (5):
t.fd(200)
t.lt(144)
t.lt(180)
t.begin_fill() #fill the 3rd star with "green"
for x in range (5):
t.fd(200)
t.rt(144)
t.end_fill()
Example 2 Circle Spiral
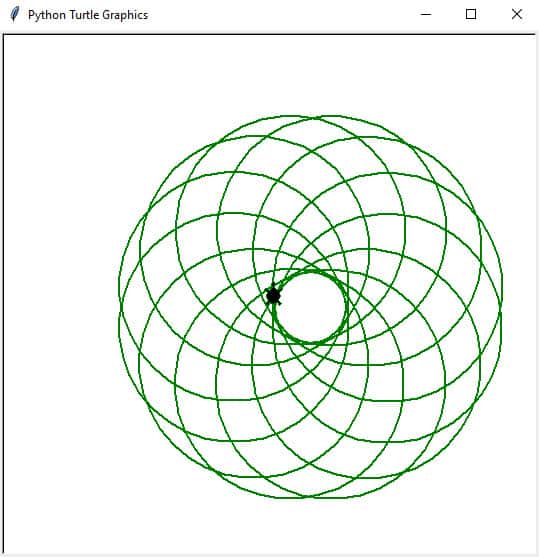
Python Turtle Code:
import turtle
t = turtle.Pen()
t.speed(0) #drawing speed
t.shape("turtle")
t.pencolor("green")
t.pensize(2)
t.lt(90) #to make sure turtle is facing north at the start
for x in range (12):
for y in range (36):
t.fd(20)
t.rt(10)
t.rt(30)
t.fd(20)
Example 3 Flower
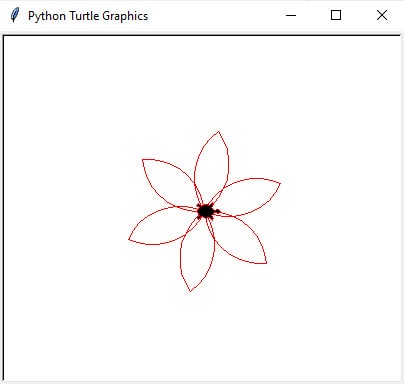
Python Turtle Code:
import turtle
t = turtle.Pen()
t.speed(0) #drawing speed
t.shape("turtle")
t.pencolor("red")
t.pensize(1)
for x in range (6):
t.rt(60) #6 pedals=> 360/6=60
for y in range (9):
t.fd(10)
t.rt(10)
t.rt(90)
for z in range (9):
t.fd(10)
t.rt(10)
t.rt(90)
What is Python Turtle? – A wrap-up
Turtle Graphic is a classical but still very applicable and useful educational tool. The pre-installed Python Turtle library offers teachers, parents, and students a fun and effective way to start learning Python programming.
Now, go ahead and draw some fancy graphics!
Download FREE Python Turtle Worksheet
I have prepared a list of 6 Python Turtle Graphic challenges for you. Each challenge comes with tips, additional challenges, and suggested solutions.
Feel free to download and share it.
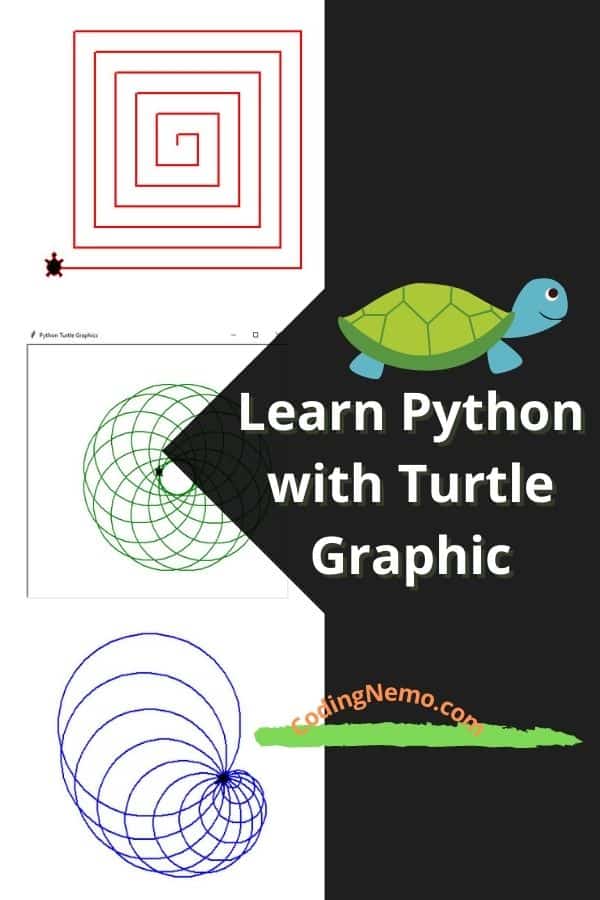
Thnx!